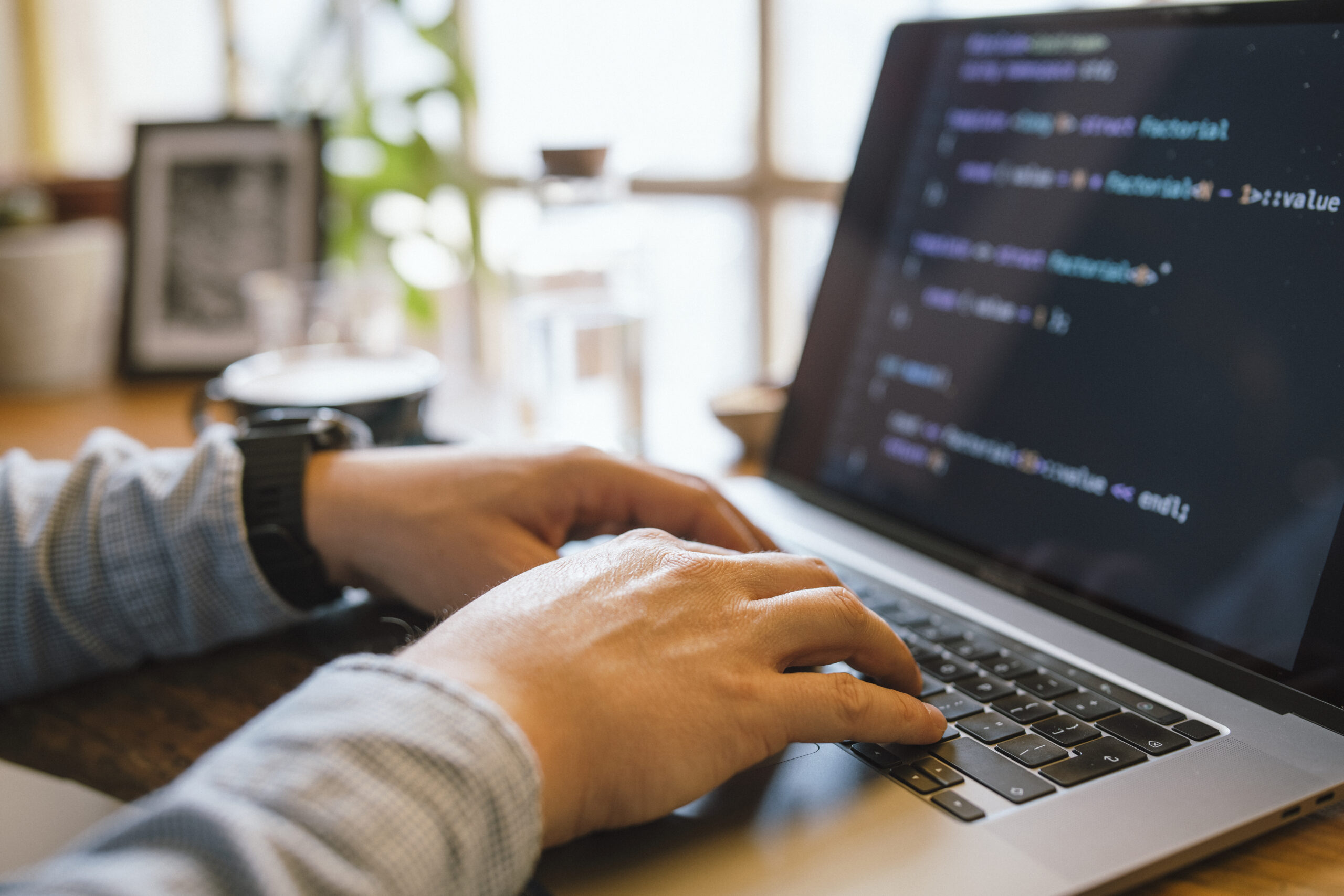
Debugging is One of the more vital — yet usually neglected — competencies in a developer’s toolkit. It isn't pretty much correcting damaged code; it’s about comprehending how and why items go Improper, and Mastering to Believe methodically to solve challenges efficiently. Whether or not you're a newbie or maybe a seasoned developer, sharpening your debugging competencies can help you save several hours of irritation and radically help your productivity. Listed here are numerous methods to aid developers degree up their debugging match by me, Gustavo Woltmann.
Grasp Your Equipment
One of several fastest approaches developers can elevate their debugging abilities is by mastering the equipment they use every day. Though producing code is one particular Portion of improvement, being aware of the best way to interact with it correctly all through execution is equally essential. Present day advancement environments come Geared up with strong debugging capabilities — but lots of builders only scratch the floor of what these resources can perform.
Just take, for instance, an Built-in Growth Setting (IDE) like Visual Studio Code, IntelliJ, or Eclipse. These instruments enable you to set breakpoints, inspect the worth of variables at runtime, phase by way of code line by line, as well as modify code over the fly. When utilised properly, they Enable you to observe precisely how your code behaves all through execution, that's invaluable for tracking down elusive bugs.
Browser developer resources, for instance Chrome DevTools, are indispensable for front-stop builders. They let you inspect the DOM, monitor network requests, view actual-time functionality metrics, and debug JavaScript in the browser. Mastering the console, resources, and network tabs can convert irritating UI difficulties into manageable responsibilities.
For backend or method-stage builders, tools like GDB (GNU Debugger), Valgrind, or LLDB supply deep Command in excess of functioning processes and memory management. Mastering these tools could have a steeper Mastering curve but pays off when debugging functionality issues, memory leaks, or segmentation faults.
Past your IDE or debugger, develop into snug with version Manage programs like Git to know code background, come across the precise instant bugs were introduced, and isolate problematic changes.
In the end, mastering your equipment signifies likely further than default settings and shortcuts — it’s about creating an personal familiarity with your enhancement environment so that when problems come up, you’re not misplaced in the dead of night. The greater you are aware of your applications, the greater time you could expend resolving the particular trouble rather then fumbling as a result of the procedure.
Reproduce the condition
One of the more crucial — and often ignored — actions in successful debugging is reproducing the issue. Prior to jumping in to the code or creating guesses, builders have to have to make a regular surroundings or situation in which the bug reliably appears. With out reproducibility, correcting a bug will become a match of likelihood, frequently leading to squandered time and fragile code modifications.
The initial step in reproducing a challenge is gathering just as much context as is possible. Check with inquiries like: What actions brought about The problem? Which atmosphere was it in — enhancement, staging, or generation? Are there any logs, screenshots, or mistake messages? The more depth you've got, the easier it will become to isolate the exact disorders underneath which the bug happens.
Once you’ve gathered sufficient information, endeavor to recreate the trouble in your neighborhood setting. This may indicate inputting the same knowledge, simulating similar consumer interactions, or mimicking system states. If The difficulty appears intermittently, look at creating automatic tests that replicate the edge scenarios or condition transitions associated. These exams not simply help expose the challenge but will also avoid regressions Down the road.
Often, The difficulty might be setting-specific — it might come about only on sure operating techniques, browsers, or less than particular configurations. Making use of instruments like Digital machines, containerization (e.g., Docker), or cross-browser screening platforms is often instrumental in replicating such bugs.
Reproducing the trouble isn’t merely a move — it’s a mindset. It needs endurance, observation, along with a methodical technique. But as you can regularly recreate the bug, you are previously midway to correcting it. Using a reproducible circumstance, You should utilize your debugging applications more successfully, check prospective fixes securely, and communicate much more clearly along with your crew or end users. It turns an abstract grievance into a concrete problem — Which’s where by builders prosper.
Read and Comprehend the Error Messages
Mistake messages are sometimes the most worthy clues a developer has when a thing goes Erroneous. In lieu of observing them as annoying interruptions, developers really should understand to deal with error messages as direct communications within the procedure. They generally tell you what exactly occurred, exactly where it happened, and sometimes even why it transpired — if you know the way to interpret them.
Start off by looking through the information thoroughly and in comprehensive. Lots of builders, especially when less than time force, look at the initial line and right away start earning assumptions. But deeper within the mistake stack or logs could lie the real root trigger. Don’t just duplicate and paste mistake messages into engines like google — go through and have an understanding of them initial.
Crack the mistake down into areas. Is it a syntax mistake, a runtime exception, or perhaps a logic mistake? Will it position to a specific file and line number? What module or operate triggered it? These inquiries can manual your investigation and place you toward the dependable code.
It’s also useful to understand the terminology on the programming language or framework you’re using. Error messages in languages like Python, JavaScript, or Java normally stick to predictable styles, and learning to recognize these can significantly hasten your debugging procedure.
Some problems are imprecise or generic, and in Individuals scenarios, it’s important to look at the context where the mistake occurred. Examine similar log entries, input values, and recent improvements in the codebase.
Don’t ignore compiler or linter warnings either. These usually precede much larger issues and provide hints about probable bugs.
Ultimately, error messages usually are not your enemies—they’re your guides. Studying to interpret them accurately turns chaos into clarity, serving to you pinpoint challenges quicker, minimize debugging time, and turn into a additional economical and assured developer.
Use Logging Correctly
Logging is Among the most impressive applications in a developer’s debugging toolkit. When employed properly, it offers real-time insights into how an application behaves, supporting you recognize what’s occurring beneath the hood while not having to pause execution or phase throughout the code line by line.
An excellent logging method starts off with recognizing what to log and at what amount. Prevalent logging degrees include things like DEBUG, Details, WARN, ERROR, and FATAL. Use DEBUG for in depth diagnostic details throughout improvement, INFO for general events (like effective start-ups), WARN for potential challenges that don’t split the appliance, ERROR for actual complications, and FATAL in the event the method can’t continue.
Stay clear of flooding your logs with abnormal or irrelevant knowledge. An excessive amount of logging can obscure important messages and decelerate your technique. Target critical functions, state changes, enter/output values, and critical conclusion factors inside your code.
Structure your log messages Obviously and persistently. Consist of context, which include timestamps, request IDs, and performance names, so it’s simpler to trace problems in dispersed methods or multi-threaded environments. Structured logging (e.g., JSON logs) will make it even much easier to parse and filter logs programmatically.
In the course of debugging, logs let you observe how variables evolve, what conditions are fulfilled, and what branches of logic are executed—all with out halting the program. They’re Specially valuable in output environments the place stepping through code isn’t doable.
In addition, use logging frameworks and instruments (like Log4j, Winston, or Python’s logging module) that support log rotation, filtering, and integration with monitoring dashboards.
Finally, sensible logging is about harmony and clarity. Using a perfectly-believed-out logging solution, you'll be able to lessen the time it takes to spot difficulties, gain further visibility into your purposes, and improve the Total maintainability and trustworthiness of your code.
Feel Just like a Detective
Debugging is not merely a technical activity—it is a type of investigation. To properly detect and fix bugs, developers have to tactic the method similar to a detective resolving a thriller. This way of thinking assists stop working elaborate concerns into workable areas and observe clues logically to uncover the foundation trigger.
Commence by collecting proof. Consider the indications of the problem: error messages, incorrect output, or general performance problems. Similar to a detective surveys a criminal offense scene, accumulate just as much suitable information and facts as you can with out jumping to conclusions. Use logs, take a look at conditions, and person stories to piece alongside one another a transparent image of what’s taking place.
Up coming, type hypotheses. Request on your own: What may very well be resulting in this habits? Have any changes lately been created on the codebase? Has this concern occurred in advance of beneath equivalent situations? The objective is to slender down opportunities and recognize possible culprits.
Then, exam your theories systematically. Attempt to recreate the condition in a very controlled atmosphere. For those who suspect a certain perform or component, isolate it and confirm if the issue persists. Similar to a detective conducting interviews, talk to your code inquiries and Allow the results guide you closer to the truth.
Pay near focus to compact information. Bugs generally conceal during the minimum expected spots—like a lacking semicolon, an off-by-1 mistake, or perhaps a race ailment. Be complete and affected person, resisting the urge to patch The difficulty without having fully comprehension it. Temporary fixes may well hide the true issue, just for it to resurface later on.
Last of all, preserve notes on Anything you experimented with and acquired. Just as detectives log their investigations, documenting your debugging course of action can conserve time for upcoming problems and enable Other folks understand your reasoning.
By pondering similar to a detective, builders can sharpen their analytical abilities, tactic problems methodically, and turn into more practical at uncovering hidden troubles in sophisticated units.
Create Assessments
Writing exams is one of the simplest tips on how to enhance your debugging capabilities and In general progress performance. Tests not merely support capture bugs early but will also function a safety Internet that provides you assurance when creating variations to your codebase. A well-tested application is simpler to debug since it lets you pinpoint particularly wherever and when a dilemma takes place.
Get started with unit assessments, which target particular person capabilities or modules. These tiny, isolated exams can speedily reveal no matter whether a certain piece of logic is Functioning as envisioned. Every time a take a look at fails, you quickly know the place to search, substantially decreasing the time spent debugging. Device assessments are Specially useful for catching regression bugs—issues that reappear just after Beforehand staying mounted.
Subsequent, combine integration assessments and conclude-to-stop tests into your workflow. These assistance be sure that various aspects of your application function together effortlessly. They’re notably beneficial for catching bugs that occur in elaborate programs with several components or solutions interacting. If a little something breaks, your assessments can let you know which Element of the pipeline failed and underneath what circumstances.
Crafting assessments also forces you to Assume critically about your code. To check a aspect appropriately, you'll need to be familiar with its inputs, anticipated outputs, and edge cases. This standard of knowing naturally qualified prospects to raised code construction and much less bugs.
When debugging a problem, writing a failing examination that reproduces the bug is usually a powerful initial step. As soon as the check fails continuously, you'll be able to center on fixing the bug and enjoy your test move when The difficulty is settled. This solution ensures that precisely the same bug doesn’t return Down the road.
In brief, producing checks turns debugging from a aggravating guessing video game right into a structured and predictable procedure—aiding you capture much more bugs, more rapidly plus more reliably.
Consider Breaks
When debugging a difficult situation, it’s uncomplicated to be immersed in the trouble—observing your monitor for hours, attempting Remedy after Answer. But The most underrated debugging instruments is actually stepping absent. Getting breaks allows you reset your intellect, reduce aggravation, and often see the issue from a new perspective.
When you're way too near to the code for way too prolonged, cognitive tiredness sets in. You could possibly start out overlooking evident glitches or misreading code that you just wrote just hrs previously. On this condition, your brain becomes significantly less productive at difficulty-solving. A brief wander, a espresso break, and even switching to a special job for 10–quarter-hour can refresh your concentration. Quite a few builders report acquiring the basis of an issue after they've taken the perfect time to disconnect, letting their subconscious work during the qualifications.
Breaks also support avoid burnout, Particularly during for a longer period debugging classes. Sitting in front of a screen, mentally trapped, is not merely unproductive but also draining. Stepping absent lets you return with renewed Power in addition to a clearer way of thinking. You could suddenly see a lacking semicolon, a logic flaw, or perhaps a misplaced variable that eluded you right before.
In case you’re caught, a good guideline would be to established a timer—debug actively for 45–sixty minutes, then take a 5–ten minute crack. Use that time to maneuver about, extend, or do some thing unrelated to code. It might sense counterintuitive, Specifically less than tight deadlines, but it surely really brings about check here quicker and simpler debugging in the long run.
In a nutshell, having breaks just isn't an indication of weak spot—it’s a smart tactic. It offers your Mind space to breathe, enhances your standpoint, and will help you steer clear of the tunnel vision That usually blocks your development. Debugging is a mental puzzle, and rest is an element of resolving it.
Discover From Every single Bug
Every bug you face is more than just A brief setback—It is really an opportunity to develop being a developer. Whether it’s a syntax error, a logic flaw, or even a deep architectural challenge, every one can teach you one thing worthwhile for those who make an effort to reflect and assess what went wrong.
Begin by asking by yourself some vital thoughts once the bug is resolved: What brought on it? Why did it go unnoticed? Could it have already been caught previously with superior techniques like device screening, code opinions, or logging? The responses often reveal blind places in your workflow or comprehending and assist you to Develop more powerful coding routines shifting forward.
Documenting bugs can also be an excellent habit. Keep a developer journal or manage a log in which you Observe down bugs you’ve encountered, the way you solved them, and Anything you figured out. After some time, you’ll start to see patterns—recurring troubles or widespread blunders—that you could proactively steer clear of.
In team environments, sharing Anything you've figured out from a bug together with your friends is often Specially effective. Whether or not it’s through a Slack message, a brief publish-up, or a quick awareness-sharing session, serving to Other individuals avoid the similar situation boosts team performance and cultivates a more powerful learning lifestyle.
Much more importantly, viewing bugs as classes shifts your attitude from frustration to curiosity. In place of dreading bugs, you’ll commence appreciating them as crucial aspects of your progress journey. In any case, a lot of the ideal builders will not be those who compose fantastic code, but people who consistently find out from their issues.
Ultimately, Each individual bug you resolve provides a brand new layer on your skill set. So next time you squash a bug, take a minute to reflect—you’ll arrive absent a smarter, more capable developer thanks to it.
Conclusion
Strengthening your debugging competencies will take time, exercise, and patience — nevertheless the payoff is big. It makes you a more productive, self-assured, and able developer. The next time you might be knee-deep in a mysterious bug, bear in mind: debugging isn’t a chore — it’s a possibility to be greater at Anything you do.